JavaScript Logical Output Based Interview Questions
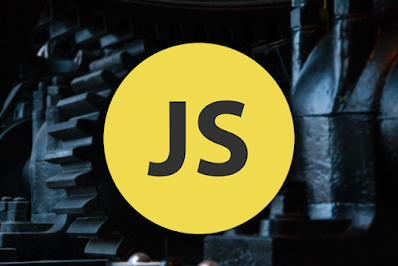
You mostly seen in JavaScript interviews there interviewers must asks output based questions in which candidate stuck mostly so here in this blog I'm telling you most commonly asked output based JavaScript interview questions. By Education Funda Most Commonly Asked JavaScript Output Based Questions Question: 1 function sum(){ let a = 8; const b=2; var c=a+b; } console.log(a) // Reference error: a is not defined console.log(b) // Reference error: b is not defined console.log(c) // Reference error: c is not defined sum() console.log(a) // Reference error: a is not defined console.log(b) // Reference error: b is not defined console.log(c) //Output: undefined ---------------------------------------------------------------------------- Question: 2 let arr=[1,2,3,4,5]; console.log(arr[2], arr.length); //Output: 3 5 arr.length=0; console.log(arr[2], arr.length); //Output: undefined 0 Note: As we can see in above code 1st ques...